After learning about the data types list
, tuple
, and set
let’s talk about the Python Dictionary (dict
). The Python dictionary is an ordered and mutable data type that stores key-value pairs. That means there is no index as you know them from lists
and tuples
; instead, there is a unique key to access an element stored in a dictionary. If you are already familiar with other programming languages, you might see a lot of similarities to associative arrays and hashmaps.
Free Python Dictionaries Cheat Sheet
Pick up your free Python dictionaries cheat sheet from my Gumroad shop:
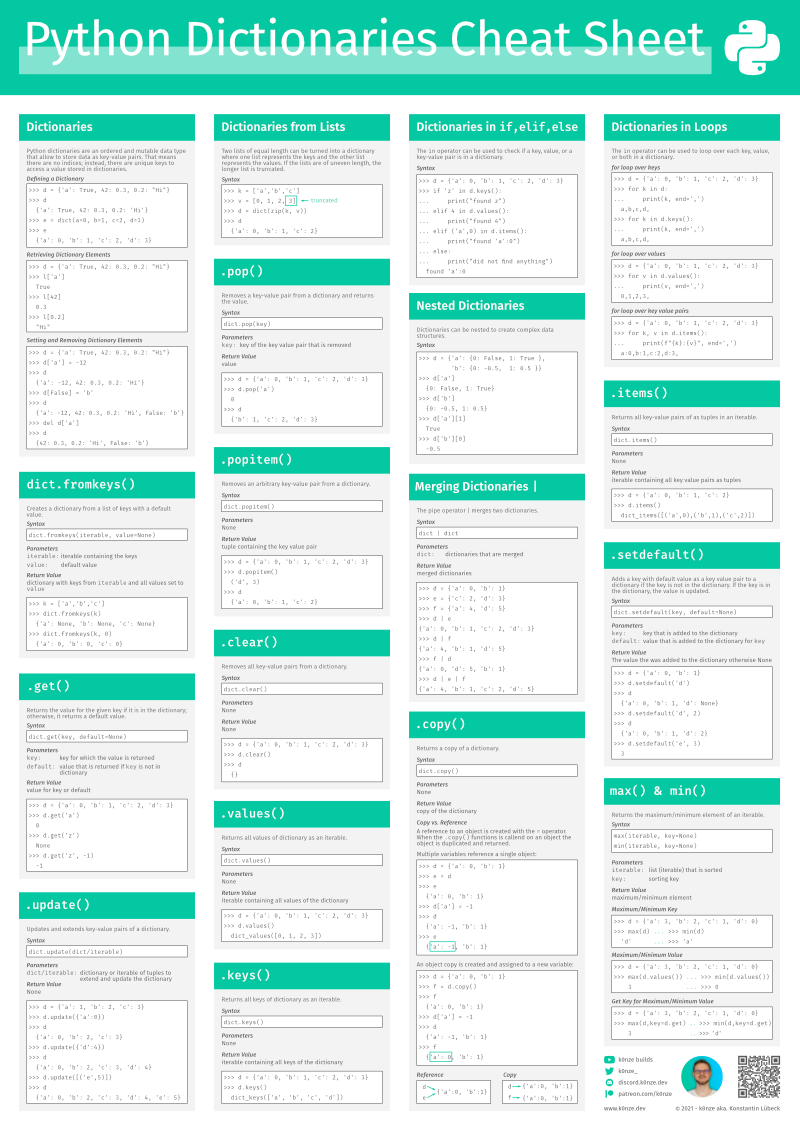
Declaring a Python Dictionary
To declare a new dictionary in Python you have to provide a key and a value which are connected with a colon :
and separated from other key value pairs by commas. All key value pairs are enclosed by braces {
}
:
1
2
3
>>> d = {'is_cat': True, 'name': "Mr. Fluffers", 'age_years': 7, 'weight_kgs': 6.1}
>>> d
{'is_cat': True, 'name': 'Mr. Fluffers', 'age_years': 7, 'weight_kgs': 6.1}
The data type of the keys and the values can be mixed. However, the keys have to be unique. Because of this, dictionaries allow you to bundle complex and structured data into a single data structure.
Python Dictionary from Lists
Another way of declaring a Python dictionary is using two lists containing the keys and values where the matching indecies of both lists are the key values pairs of the dictionary that is created. To match the indecies of both lists the zip()
function is used and than passed to the dict()
function:
1
2
3
4
5
>>> keys = ['is_cat', 'name', 'age_years', 'weight_kgs']
>>> values = [True, "Mr. Fluffers", 7, 6.1]
>>> d = dict(zip(keys, values))
>>> d
{'is_cat': True, 'name': 'Mr. Fluffers', 'age_years': 7, 'weight_kgs': 6.1}
If you don’t want to set the values when creating a new dictionary, you already know the keys use the .fromkeys()
function. The .fromkeys()
function takes a list of keys and creates a dictionary where all values are set to None
1
2
3
>>> keys = ['is_cat', 'name', 'age_years', 'weight_kgs']
>>> dict.fromkeys(keys)
{'is_cat': None, 'name': None, 'age_years': None, 'weight_kgs': None}
It is also possible to pass a different default for the values by passing a second parameter the the .fromkeys()
function:
1
2
3
>>> keys = ['is_cat', 'name', 'age_years', 'weight_kgs']
>>> dict.fromkeys(keys, 0)
{'is_cat': 0, 'name': 0, 'age_years': 0, 'weight_kgs': 0}
Accessing Python Dictionary Elements
There are several ways to access the data stored in a dictionary. The first and easiest way is the bracket operator []
. To access a value from a Python dictionary with the []
-operator, the key for the value is placed between the brackets. However, the key has to exist; otherwise, it results in a KeyError
:
1
2
3
4
5
6
7
8
9
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d['a']
1
>>> d['b']
2
>>> d['z']
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
KeyError: 'z'
To avoid the KeyError
you can use the .get()
function to access values with keys. The .get()
function takes the key as a parameter and returns the value for that key. If the key does not exist it returns None
by default but you can also set your own default by passing another parameter after the key:
1
2
3
4
5
6
7
8
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.get('a')
1
>>> d.get('b')
2
>>> d.get('z')
>>> d.get('z', -1)
-1
The .items()
function allows you to access the key values pairs as tuple
s in a list
:
1
2
3
4
5
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> l = list(d.items())
[('a', 1), ('b', 2), ('c', 3)]
>>> l[0]
('a', 1)
Adding new Elements to a Python Dictionary
To add a new key value pair or update an existing one to a Python dictionary you can also use the []
-operator and enter the key to update or add and follow it with a =
and the value:
1
2
3
4
5
6
7
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d['a'] = 0
>>> d
{'a': 0, 'b': 2, 'c': 3}
>>> d['d'] = 4
>>> d
{'a': 0, 'b': 2, 'c': 3, 'd': 4}
Python also provides a dedicated function to add or update a key value pair with the .update()
function. The .update()
function either takes another dictionary as an argument or a list
of 2-tuple
s representing key value pairs:
1
2
3
4
5
6
7
8
9
10
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.update({'a':0})
>>> d
{'a': 0, 'b': 2, 'c': 3}
>>> d.update({'d':4})
>>> d
{'a': 0, 'b': 2, 'c': 3, 'd': 4}
>>> d.update([('e',5)])
>>> d
{'a': 0, 'b': 2, 'c': 3, 'd': 4, 'e': 5}
Removing Elements from a Python Dictionary
With the .pop()
function a key value pair is removed from a dictionary and returns the value when passing the key as a parameter:
1
2
3
4
5
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.pop('a')
1
>>> d
{'b': 2, 'c': 3}
The .popitem()
function has no parameters and removes an arbitrary key value pair from a dictionary and returns it as a 2-tuple
:
1
2
3
4
5
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.popitem()
('c', 3)
>>> d
{'a': 1, 'b': 2}
To remove all elements from a dictionary and end up with an empty dictionary use the .clear()
function:
1
2
3
4
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.clear()
>>> d
{}
Merging Python Dictionaries
When you got two dictionaries and want to merge them into one use the pipe (|
) operator. If the two dictionaries that should be merged don’t have the same keys you get a dictionary containing all key value pairs of both dictionaries. However, when a key is present in both dictionaries the value of the second dictionary is merged into the new one:
1
2
3
4
5
6
7
8
9
>>> d = {'a': 1, 'b': 2}
>>> e = {'c': 3, 'd': 4}
>>> d | e
{'a': 1, 'b': 2, 'c': 3, 'd': 4}
>>> f = {'b': -1, 'c': 3}
>>> d | f
{'a': 1, 'b': -1, 'c': 3}
>>> f | d
{'b': 2, 'c': 3, 'a': 1}
Nested Python Dictionaries
One of Python dictionaries’ most potent properties is the ability to nest them such that the values are dictionaries themselves. This allows you to build complex data structures. For Example, suppose you got several pets and want to store information about them structured. In that case, you could use nested Python dictionaries and use stacked []
-operators to access and update the data:
1
2
3
4
5
6
7
>>> pets = {'Mr. Fluffers': {'species': 'cat', 'age_years': 7, 'weight_kgs': 6.1},
... 'Rambo': {'species': 'dog', 'age_years': 4, 'weight_kgs': 31 }}
>>> pets['Mr. Fluffers']['species']
'cat'
>>> pets['Rambo']['age_years'] = 5
>>> pets
{'Mr. Fluffers': {'species': 'cat', 'age_years': 7, 'weight_kgs': 6.1}, 'Rambo': {'species': 'dog', 'age_years': 5, 'weight_kgs': 31}}
Python Dictionary Functions
Python provides additional functions to access and manipulate dictionaries.
.values()
The .values()
function returns all values in a dictionary:
1
2
3
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.values()
dict_values([1, 2, 3])
Together with in
operator you can check if a specific value is contained in a dictionary:
1
2
3
4
5
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> 1 in d.values()
True
>>> 4 in d.values()
False
.keys()
Similarly to the .values()
function is the .keys()
function which returns all the keys of a dictionary:
1
2
3
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.keys()
dict_keys(['a', 'b', 'c'])
And with the in
operator you can check if a key is contained in a dictionary:
1
2
3
4
5
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> 'a' in d.keys()
True
>>> 'z' in d.keys()
False
You actually don’t need the .keys()
function to check the existence of a key in dictionary you can just use in
and it will call .keys()
by default:
1
2
3
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> 'a' in d
True
However, I suggest you use .keys()
to make your code more readable.
.setdefault()
With the .setdefault()
function you can insert a key with the None
value into a dictionary if the key doesn’t exist yet. If the key is already present in the dictionary .setdefault()
returns the value:
1
2
3
4
5
6
7
8
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.setdefault('z')
>>> d
{'a': 1, 'b': 2, 'c': 3, 'z': None}
>>> d.setdefault('a')
1
>>> d
{'a': 1, 'b': 2, 'c': 3, 'z': None}
You can also change the default value by passing a second parameter after the key:
1
2
3
4
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> d.setdefault('z', -1)
>>> d
{'a': 1, 'b': 2, 'c': 3, 'z': -1}
.copy()
Python dictionaries are mutable and therefore they provide a .copy()
function which allows you to create a copy of dictionary:
1
2
3
4
5
6
7
8
9
10
>>> d = {'a': 1, 'b': 2, 'c': 3}
>>> e = d
>>> f = d.copy()
>>> e['a'] = 0
>>> e
{'a': 0, 'b': 2, 'c': 3}
>>> d
{'a': 0, 'b': 2, 'c': 3}
>>> f
{'a': 1, 'b': 2, 'c': 3}
The .copy()
function concludes this article on Python dictionaries. Make sure to get the free Python Sets Cheat Sheet in my Gumroad shop. If you have any questions about this article, feel free to join our Discord community to ask them over there.