In this article, you will learn about the sequential data type list
. In the first article on data types you have seen the primitive data types bool
, int
, float
and str
. While str
is also a sequential data type and behaves similar to a list
in many ways with one key difference. A str
can only store values of one kind, and those are characters such as letters and numbers. list
s however, can store other types and each element can be of a different data type.
Free Python Lists Cheat Sheet
Pick up you free Python lists cheat sheet from my Gumroad shop:
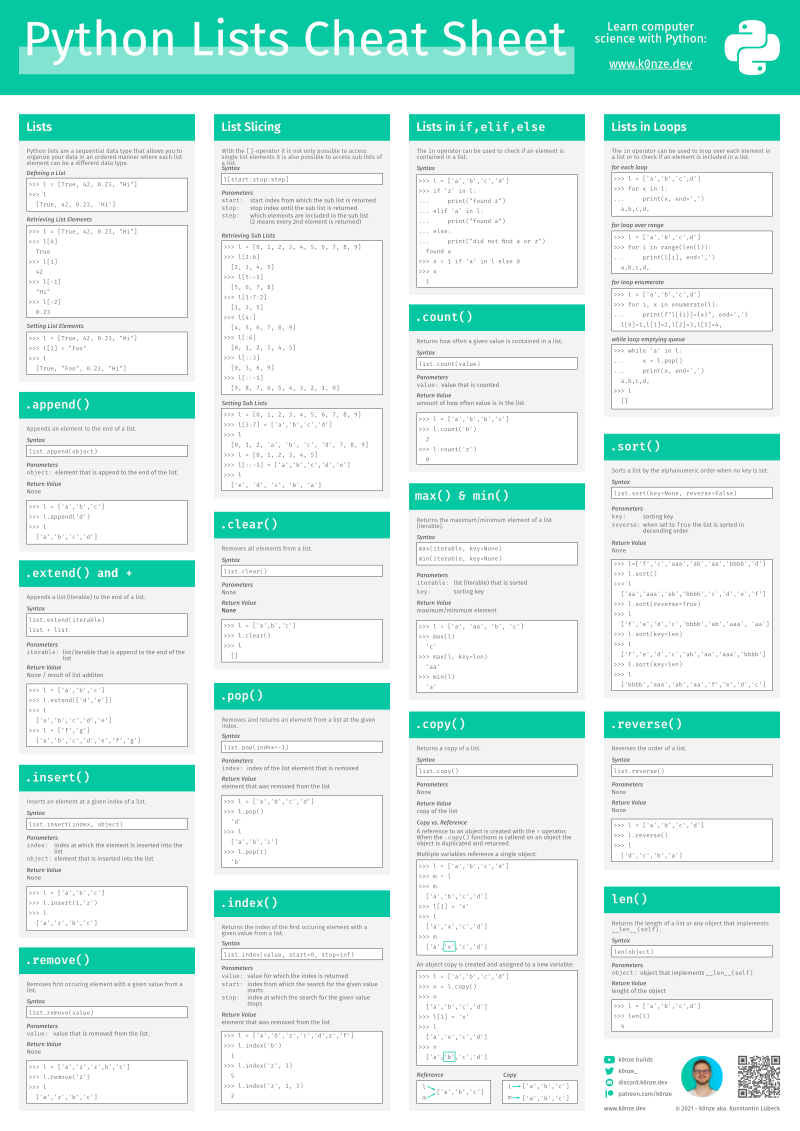
Declaring a Python List
To declare a list
you put all the values of a list
between two brackets []
and separate them with commas ,
. In the following example, the list containing a bool
, int
, float
, and str
is assigned to the variable l
:
1
2
3
>>> l = [True, 42, 0.23, "Hi"]
>>> l
[True, 42, 0.23, 'Hi']
Accessing Python List Elements
You can either access the whole list by stating the variable l
or you can access individual elements with the []
-operator (also called bracket-operator). Each element of a list
has an index starting from the left with 0, adding 1 in every step going to the right.
To access the first element, you need to enter a 0
as an index between the brackets directly added after the variable name. For the l
declared above, you would get True
as a value:
1
2
>>> l[0]
True
To access the second element, you replace 0
with a 1
and so on. But how would you access the last element or the second last element? One solution is to use len(...)
. len(...)
returns the length of a list
as an int
:
1
2
>>> len(l)
4
The last element of a list is always at index len(...)-1
. However, Python has a trick up its sleeve, so you don’t have to use len(...)
; instead, you can use negative indices. The index -1
will always return the last element of a list, and the index -2
returns the second last element and so on.
1
2
3
4
>>> l[-1]
'Hi'
>>> l[-2]
0.23
Python List Slicing
With the bracket-operator it is also possible to get a sub list
that represents only a part of the original list. This is called list
slicing. To make the examples a bit easier to comprehend, declare a list ` i’ where the elements have the same value as the index:
1
>>> i = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 16, 17, 18, 19]
If now want to slice out the list
[4, 5, 6, 7, 8]
you enter the following:
1
2
>>> i[4:9]
[4, 5, 6, 7, 8]
The first index (here 4
) is the start index. From this index on, all elements are returned one position before the second index (here 9
). If you want a list
starting from a certain index until the end of the list
, you don’t have to enter a second index:
1
2
>>> i[13:]
[13, 14, 16, 17, 18, 19]
And if you want a list
from the beginning up to a certain index, don’t enter the first index:
1
2
>>> i[:7]
[0, 1, 2, 3, 4, 5, 6]
It is possible to only get every n-th element of a list
with list
slicing by adding another colon :
after the second index indicating the step width. If you only want every 3rd element of the sub list
i[5:18]
use the following:
1
2
>>> i[5:18:3]
[5, 8, 11, 14, 18]
And as before, you can leave out the first and second index or both if the end of your sub list
should be the start or end of the original list:
1
2
>>> i[::3]
[0, 3, 6, 9, 12, 16, 19]
Variables in Python Lists
You can also use variables to add elements to a list. However, the values stored in the variable will be copied over into the list element, which means when you change the value of a variable after it has been added to a list, the change won’t appear in the list:
1
2
3
4
5
6
7
8
9
>>> a = 12
>>> b = True
>>> c = 0.21
>>> l = [a, b, c]
>>> l
[12, True, 0.21]
>>> a = 4
>>> l
[12, True, 0.21]
A list can also contain other lists:
1
2
3
4
5
>>> m = [3, 4, 5]
>>> n = [6, 7, 8]
>>> l = [m, n]
>>> l
[[3, 4, 5], [6, 7, 8]]
This can be helpful if you want to represent your data in a table, for example. To access the 1st element of the 2nd list
, you stack the []
-operator:
1
2
>>> l[1][0]
6
The first bracket-operator [1]
accesses the outer list
and returns the element stored and this index 1
which is the inner list [6, 7, 8]
. The second bracket-operator now acts on the list
returned by the first bracket-operator and returns the element at index 0
.
You are also able to use variables as index with in the brackets:
1
2
3
4
5
6
>>> i = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 16, 17, 18, 19]
>>> a = 1
>>> b = 12
>>> c = 4
>>> i[a:b:c]
[1, 5, 9]
Changing Python List Elements
The []
-operator can also be used to assign new values to elements in a list
:
1
2
3
4
>>> l = ["Hello", "World", "!"]
>>> l[2] = "?"
>>> l
['Hello', 'World', '?']
And you can even bulk-assign new values to list elements using list
slicing:
1
2
3
4
>>> i = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 16, 17, 18, 19]
>>> i[1:7:2] = [10,30,50]
>>> i
[0, 10, 2, 30, 4, 50, 6, 7, 8, 9, 10, 11, 12, 13, 14, 16, 17, 18, 19]
But you have to be careful here because the selected slice and the list containing the new values have to be the same size to make this bulk-assignment work.
Python Mutable Data Types
Changing the values stored in list
elements is a property of a mutable data type. A mutable data types also allow you to add and remove elements after it is declared. In contrast to mutable data, types are immutable ones where you aren’t able to add or remove elements after their declaration. You will see an example of an immutable data type in an upcoming article on Python tuples
.
Now let’s take a close look at how to add, remove, and manipulate list
s in Python
Python List Manipulation Functions
A list
manipulation function is always called with a dot .
after the list
variable name and then stating the function’s name followed by parenthesis with the function’s parameters.
.append()
To add (or append) a single element to the end of a list
use the append()
function:
1
2
3
4
>>> l = ['a', 'b', 'c']
>>> l.append('d')
>>> l
['a', 'b', 'c', 'd']
.extend() or +
If you want to append several elements (a list
of elements) to the end of a list
use extend()
or +
:
1
2
3
4
5
6
7
>>> l = ['a', 'b', 'c']
>>> l.extend(['d', 'e'])
>>> l
['a', 'b', 'c', 'd', 'e']
>>> l = l + ['f', 'g']
>>> l
['a', 'b', 'c', 'd', 'e', 'f', 'g']
.insert()
To add a new element to a list at a certain index of a list
while moving all current elements one index further back use insert()
where the first parameter of index()
is the index at which you would like to insert the new element and the second parameter is the element to insert itself. And don’t forget that the first index of a Python list
is 0
:
1
2
3
4
>>> l = ['a', 'b', 'c']
>>> l.insert(1, 'z')
>>> l
['a', 'z', 'b', 'c']
.remove()
To remove an element from a list
use the remove()
function which will remove the first matching element in your list
going from left to right:
1
2
3
4
>>> l = ['a', 'z', 'z', 'b', 'c']
>>> l.remove('z')
>>> l
['a', 'z', 'b', 'c']
.clear()
If you want to remove all elements from a list
and end up with an empty list use clear()
:
1
2
3
4
>>> l = ['a', 'b', 'c']
>>> l.clear()
>>> l
[]
.index()
To figure out the index of a list
element use the index()
function which tells you the index of the first matching element in your list
going from left to right:
1
2
3
>>> l = ['a', 'z', 'z', 'b', 'c']
>>> l.index('z')
1
.pop()
Removing a list
element from an arbitrary index can be done using the pop()
function. pop()
also returns the the element that should be removed. When passing no index to pop()
the last list element will be removed and returned:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
>>> l = ['a', 'b', 'c', 'd', 'e', 'f']
>>> l.pop()
'f'
>>> l
['a', 'b', 'c', 'd', 'e']
>>> l.pop(0)
'a'
>>> l
['b', 'c', 'd', 'e']
>>> l.pop(2)
'd'
>>> l
['b', 'c', 'e']
>>>
.sort()
You don’t even have to implement a sorting algorithm when using Python list
s. list
s come with their own sorting function sort()
. The list
is sorted alphanumerically when no parameters are passed to sort()
. You can also specify that your list
should be sorted in the reversed order by passing reverse=True
to sort()
. And if you rather want to sort a list
by the str
length of the elements, you can pass key=len
as an argument:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
>>> l = ['f', 'c', 'aaa', 'ab', 'aa', 'bbbb', 'd', 'e']
>>> l.sort()
>>> l.sort(reverse=True)
>>> l = ['f', 'c', 'aaa', 'ab', 'aa', 'bbbb', 'd', 'e']
>>> l.sort()
>>> l
['aa', 'aaa', 'ab', 'bbbb', 'c', 'd', 'e', 'f']
>>> l.sort(reverse=True)
>>> l
['f', 'e', 'd', 'c', 'bbbb', 'ab', 'aaa', 'aa']
>>> l.sort(key=len)
>>> l
['f', 'e', 'd', 'c', 'ab', 'aa', 'aaa', 'bbbb']
>>> l.sort(reverse=True, key=len)
>>> l
['bbbb', 'aaa', 'ab', 'aa', 'f', 'e', 'd', 'c']
.reverse()
To simply reverse your list use reverse()
:
1
2
3
4
>>> l = ['a', 'b', 'c', 'd', 'e', 'f']
>>> l.reverse()
>>> l
['f', 'e', 'd', 'c', 'b', 'a']
Python List Property Functions
The following functions do not manipulate the list
content. However, they are pretty helpful when you want to figure out specific properties of your list
s
len(list)
As you have already seen above when learning about the []
-operator len()
will return the length of a list:
1
2
3
>>> l = ['a', 'b', 'c']
>>> len(l)
3
.count()
If you want to know how often an element is included in a list
use count()
:
1
2
3
4
5
>>> l = ['a', 'a', 'b', 'c']
>>> l.count('a')
2
>>> l.count('x')
0
in
To check if an element is inside a list
you can also use Python’s in
operator:
1
2
3
4
5
>>> l = ['a', 'b', 'c']
>>> 'b' in l
True
>>> 'd' in l
False
max(list) and min(list)
To find the maximum and minimum element of a list
use max()
and min()
. When not passing any parameters to max()
and min()
they will return the maximum/minimum element of the alphanumeric order, however, you can set a key
such as key=len
to find the maximum/minimum element according to that key:
1
2
3
4
5
6
7
>>> l = ['a', 'aa', 'b', 'c']
>>> max(l)
'c'
>>> max(l, key=len)
'aa'
>>> min(l)
'a'
.copy()
When you want to duplicate a list
you have to sure if you want a reference or a copy. You can reference a list
by assigning the original variable to another variable, than both variables point to the same list (you could also say share a list
). When referencing a list
a change using one variable will also be reflected when accessing the other variable.
If you need want to keep the original list
and only make changes in the list assigned to another variable use the .copy()
function:
1
2
3
4
5
6
7
8
9
10
>>> l = ['a', 'b', 'c']
>>> m = l
>>> n = l.copy()
>>> l[1] = 'x'
>>> l
['a', 'x', 'c']
>>> m
['a', 'x', 'c']
>>> n
['a', 'b', 'c']
The copy()
function concludes this video. Make sure to get the free Python Lists Cheat Sheet in my Gumroad shop. If you have any questions about this article, feel free to join our Discord community to ask them over there.